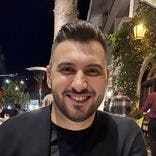
Mark Khuzam
Oct 27, 2020
5 Ways Material UI Themes Make Styling Easier
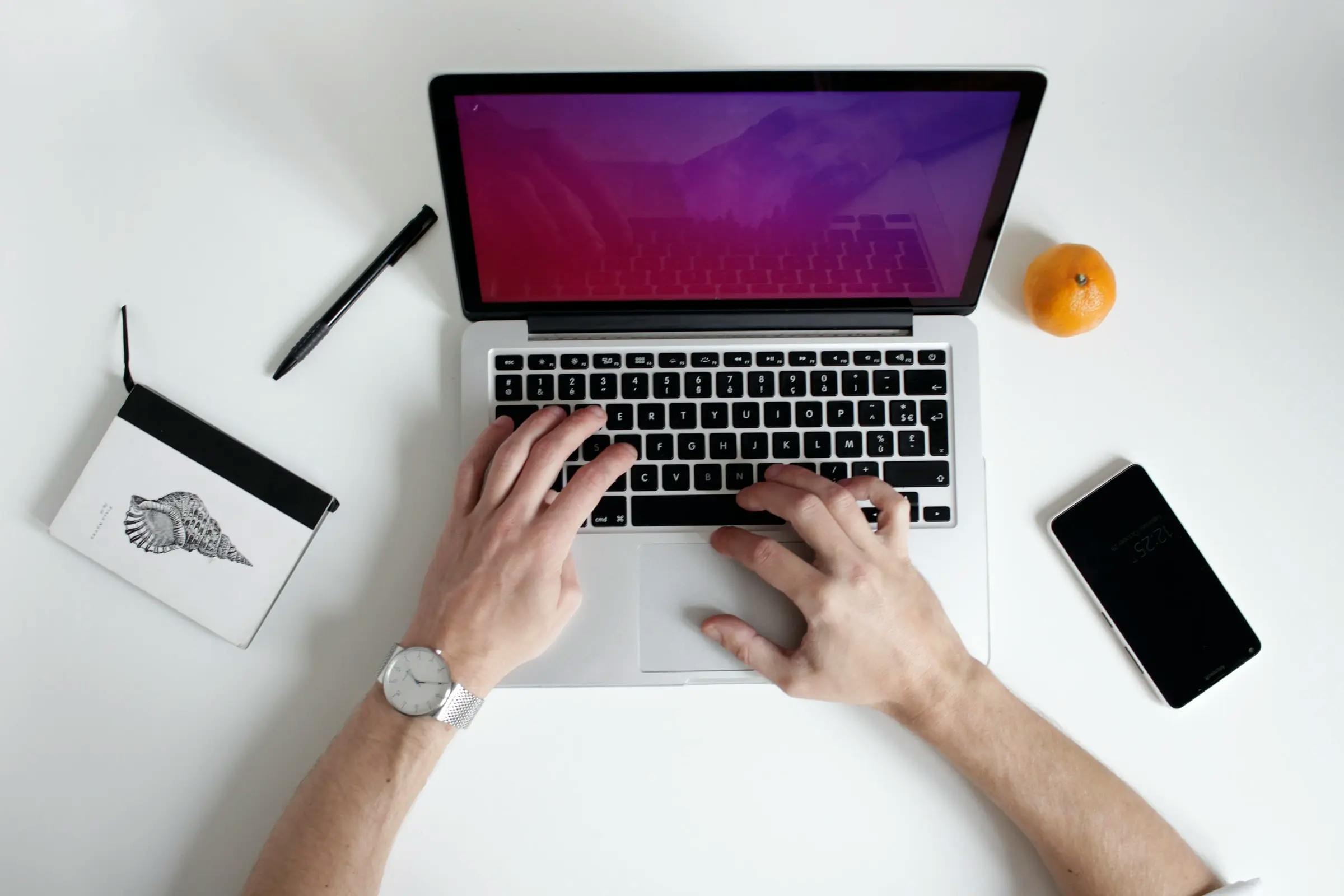
When it comes to React Component libraries, Material UI has got it all. The components look great and work well with all kinds of custom interactions. They're easily customizable and can save tons of time on styling and basic component configuration.
What I love most about the Material library is its styling solution!
The MUI styling solution provides a way to build dynamic UIs that's efficient, elegant, and scalable. It's influenced by many other libraries you may be familiar with, such as SASS/SCSS and styled-components.
Combining the styling solution with a theme gives you the ultimate setup for keeping a consistent style across colors, fonts, spacing, and responsive layouts. Here are 5 ways I use Material UI to develop faster.
1. Responsive Breakpoints and Containers for Layouts
MUI allows you to set values that determine breakpoints on the page.
Wrapping a page with a Container also allows you to set the maxWidth contents in that container are allowed to expand to given a value.
Along with responsive sizing and easy to set dimensions, Containers make sure padding and margins are consistent throughout components within the app. They're great to use with your main layout on every page.
For example, the main layout of markk.dev looks something like...
import { Container } from '@material-ui/core';
const Layout = ({ children }) => {
return (
<Container maxWidth="lg">
<Header />
{children}
<Footer />
</Container>
);
};
export default Layout;
2. Styling Components With Hooks
Let's face it, once you start using React Hooks, you never go back. Styling with hooks is awesome for a couple of reasons.
- Scalable: Each component file contains its own styles
- Dynamic: Passing props to a makeStyles hook allows you to dynamically choose styles based on values
- Theme: Hooks allow you to access your main theme and keep consistent styling throughout all components
import { makeStyles, Box } from '@material-ui/core'
const useStyles = makeStyles((theme) => {
wrapper: {
backgroundColor: (props) => props.isEven ? 'red' : 'blue',
display: 'flex',
flexDirection: 'column',
color: theme.palette.primary.main,
width: theme.spacing(20),
height: theme.spacing(20)
}
})
const RedBlueBox = ({isEven}) => {
const classes = useStyles({isEven})
return <Box className={classes.wrapper}>
...
</Box>
}
3. Consistent Spacing Everywhere
This plays into themeing and using the makeStyles hook as well but I wanted to emphasize it because it's one of the main things that I feel helps a User Interface look cohesive and well laid out.
My favorite settings for spacing are the default settings but you can also add custom spacing settings if you'd like. I won't get into that but you can reference the spacing documentation.
Back to our style from before, theme.spacing allows us to choose a numeric value that's a multiple of 8.
const useStyles = makeStyles((theme) => {
wrapper: {
width: theme.spacing(20); // = 8 * 20 = 160,
height: theme.spacing(25); // = 8 * 25= 200
}
});
4. Responsive Font Sizes and Typography
The latter half of this might only save you 1 second of time, but nonetheless, every second is precious.
Responsive Font Sizes are great because MUI will adapt whatever Font Sizes you've selected for Typography components to fit to the screen size.
import { createMuiTheme, responsiveFontSizes } from '@material-ui/core/styles';
let theme = createMuiTheme({
typography: {
h1: {
fontSize: 48
}
}
});
theme = responsiveFontSizes(theme);
And after setting your theme font sizes, you're ready to go with the Typography component and API.
const Header = () => {
return <Typography variant="h1">This is my header!</Typography>;
};
Your header text size will look good on Desktop, Tablet, and Mobile, while also containing the proper H1 SEO tag for screen readers and web crawlers.
5. No More Media Queries! (kinda)
Last but definitely not least is how easy it is to create responsive components with the breakpoints API. I touched on breakpoints earlier for how useful they are for layouts, but when you get into creating very specific components breakpoints become your best friend.
In this next example, you have a component that's originally horizontal. The problem is the width is too large for a mobile phone.
The answer is definitely to add a media query that changes the orientation of the components children when the screen size is below our predefined size.
import { makeStyles, Box } from '@material-ui/core'
const useStyles = makeStyles((theme) => {
wrapper: {
display: 'flex',
flexDirection: 'row',
[theme.breakpoints.down('xs')]:{
flexDirection: 'column'
}
}
})
const FirstRowThenColumnWrapper = () => {
return <Box className={classes.wrapper}>
...
</Box>
}
This list is a very short preview of how I use the Material UI styling solution daily. I highly encourage you to spend some time playing around with Material UI and customizing the components to fit your unique style!
Thanks for getting this far, If you haven't yet, subscribe for updates on my next blog!